HTTP ์์ฒญ ๋ฉ์์ง - ๋จ์ ํ ์คํธ
์์ฒญ ํ๋ผ๋ฏธํฐ์ ๋ค๋ฅด๊ฒ, HTTP ๋ฉ์์ง ๋ฐ๋๋ฅผ ํตํด ๋ฐ์ดํฐ๊ฐ ์ง์ ๋์ด์ค๋ ๊ฒฝ์ฐ๋
@RequestParam , @ModelAttribute ๋ฅผ ์ฌ์ฉํ ์ ์๋ค.
InputStream
ServletInputStream inputStream = request.getInputStream();
String messageBody = StreamUtils.copyToString(inputStream, StandardCharsets.UTF_8);
log.info("messageBody={}",messageBody);
HTTP ๋ฉ์์ง ๋ฐ๋์ ๋ฐ์ดํฐ๋ฅผ InputStream ์ ์ฌ์ฉํด์ ์ง์ ์ฝ์ ์ ์๋ค.
@PostMapping("/request-body-string-v2")
public void requestBodyString(InputStream inputStream, Writer responseWriter) throws IOException {
String messageBody = StreamUtils.copyToString(inputStream, StandardCharsets.UTF_8);
log.info("messageBody={}",messageBody);
responseWriter.write("OK");
InputStream(Reader): HTTP ์์ฒญ ๋ฉ์์ง ๋ฐ๋์ ๋ด์ฉ์ ์ง์ ์กฐํ
OutputStream(Writer): HTTP ์๋ต ๋ฉ์์ง์ ๋ฐ๋์ ์ง์ ๊ฒฐ๊ณผ ์ถ๋ ฅ
HttpEntity
@PostMapping("/request-body-string-v3")
public HttpEntity<String> requestBodyStringV3(HttpEntity<String> httpEntity) throws IOException {
String messageBody = httpEntity.getBody();
log.info("messageBody={}",messageBody);
return new HttpEntity<>("OK");
}
HTTP header, body ์ ๋ณด๋ฅผ ํธ๋ฆฌํ๊ฒ ์กฐํ / ๋ฉ์์ง ๋ฐ๋ ์ ๋ณด๋ฅผ ์ง์ ์กฐํ
@RequestBody / @ResponseBody
@ResponseBody
@PostMapping("/request-body-string-v4")
public String requestBodyStringV4(@RequestBody String messageBody){
log.info("messageBody={}",messageBody);
return "OK";
}
@RequestBody : HTTP ๋ฉ์์ง ๋ฐ๋๋ฅผ ์ง์ ์กฐํํ๋ ๊ธฐ๋ฅ
@ResponseBody : ์๋ต ๊ฒฐ๊ณผ๋ฅผ HTTP ๋ฉ์์ง ๋ฐ๋์ ์ง์ ๋ด์์ ์ ๋ฌ
์์ฒญ ํ๋ผ๋ฏธํฐ๋ฅผ ์กฐํํ๋ ๊ธฐ๋ฅ: @RequestParam , @ModelAttribute
HTTP ๋ฉ์์ง ๋ฐ๋๋ฅผ ์ง์ ์กฐํํ๋ ๊ธฐ๋ฅ : @RequestBody
HTTP ์์ฒญ ๋ฉ์์ง - JSON
ServletInputStream inputStream = request.getInputStream();
String messageBody = StreamUtils.copyToString(inputStream, StandardCharsets.UTF_8);
HttpServletRequest๋ฅผ ์ฌ์ฉํด์ ์ง์ HTTP ๋ฉ์์ง ๋ฐ๋์์ ๋ฐ์ดํฐ๋ฅผ ์ฝ์ด์์, ๋ฌธ์๋ก ๋ณํํ๋ค.
private ObjectMapper objectMapper = new ObjectMapper();
HelloData helloData = objectMapper.readValue(messageBody, HelloData.class);
๋ฌธ์๋ก ๋ JSON ๋ฐ์ดํฐ๋ฅผ Jackson ๋ผ์ด๋ธ๋ฌ๋ฆฌ์ธ objectMapper ๋ฅผ ์ฌ์ฉํด์ ์๋ฐ ๊ฐ์ฒด๋ก ๋ณํํ๋ค.
JSON ํ์ผ์ Java ๊ฐ์ฒด๋ก deserialization ํ๊ธฐ ์ํด์๋ ObjectMapper์ readValue() ๋ฉ์๋๋ฅผ ์ด์ฉํ๋ค.
๋ฌธ์๋ก ๋ JSON ๋ฐ์ดํฐ์ธ messageBody ๋ฅผ objectMapper ๋ฅผ ํตํด์ ์๋ฐ ๊ฐ์ฒด๋ก ๋ณํํ๋ค.
# ์ฐธ๊ณ : ObjectMapper (JSON ํ์ฑ)
ObjectMapper
ObjectMapper๋ฅผ ์ด์ฉํ๋ฉด JSON์ Java ๊ฐ์ฒด๋ก ๋ณํ
๋ฐ๋๋ก Java ๊ฐ์ฒด๋ฅผ JSON ๊ฐ์ฒด๋ก serialization ํ ์ ์๋ค.
Java Object → JSON
Java ๊ฐ์ฒด๋ฅผ JSON์ผ๋ก serialization ํ๊ธฐ ์ํด์๋ ObjectMapper์ writeValue() ๋ฉ์๋๋ฅผ ์ด์ฉํ๋ค.
// Java Object -> JSON
Person person = new Person("zooneon", 25, "seoul");
try {
objectMapper.writeValue(new File("src/person.json"), person);
} catch (IOException e) {
e.printStackTrace();
}
์์ ๊ฐ์ด ํ๋ผ๋ฏธํฐ๋ก JSON์ ์ ์ฅํ ํ์ผ๊ณผ ์ง๋ ฌํ์ํฌ ๊ฐ์ฒด๋ฅผ ๋ฃ์ด์ฃผ๋ฉด ๋๋ค.
JSON → Java Object
JSON ํ์ผ์ Java ๊ฐ์ฒด๋ก deserialization ํ๊ธฐ ์ํด์๋ ObjectMapper์ readValue() ๋ฉ์๋๋ฅผ ์ด์ฉํ๋ค.
// JSON -> Java Object
String json = "{\"name\":\"zooneon\",\"age\":25,\"address\":\"seoul\"}";
try {
Person deserializedPerson = objectMapper.readValue(json, Person.class);
System.out.println(deserializedPerson);
} catch (JsonProcessingException e) {
e.printStackTrace();
}
์์ ๊ฐ์ด ํ๋ผ๋ฏธํฐ๋ก JSON ํํ์ ๋ฌธ์์ด or ๊ฐ์ฒด์ ์ญ์ง๋ ฌํ ์ํฌ ํด๋์ค๋ฅผ ๋ฃ์ด์ฃผ๋ฉด ๋๋ค.
@RequestBody ๊ฐ์ฒด ๋ณํ
@ResponseBody
@PostMapping("request-body-json-v3")
public String requestBodyJsonV3(@RequestBody HelloData helloData) throws IOException {
log.info("username={}, age={}",helloData.getUsername(),helloData.getAge());
return "OK";
}
@RequestBody ์ ์ง์ ๋ง๋ ๊ฐ์ฒด๋ฅผ ์ง์ ํ ์ ์๋ค.
@RequestBody๋ ์๋ต ๋ถ๊ฐ๋ฅ
HelloData์ @RequestBody ๋ฅผ ์๋ตํ๋ฉด @ModelAttribute ๊ฐ ์ ์ฉ๋์ด๋ฒ๋ฆฐ๋ค.
๋ฐ๋ผ์ ์๋ตํ๋ฉด HTTP ๋ฉ์์ง ๋ฐ๋๊ฐ ์๋๋ผ ์์ฒญ ํ๋ผ๋ฏธํฐ๋ฅผ ์ฒ๋ฆฌํ๊ฒ ๋๋ค.
@ModelAttribute
์์ฒญ ํ๋ผ๋ฏธํฐ๋ฅผ ๋ฐ์์ ํ์ํ ๊ฐ์ฒด๋ฅผ ๋ง๋ค๊ณ ๊ทธ ๊ฐ์ฒด์ ๊ฐ์ ๋ฃ์ด์ค๋ค
์์ฒญ ํ๋ผ๋ฏธํฐ์ ์ด๋ฆ์ผ๋ก HelloData ๊ฐ์ฒด์ ํ๋กํผํฐ๋ฅผ ์ฐพ๋๋ค.
@ResponseBody
@ResponseBody
@PostMapping("request-body-json-v5")
public HelloData requestBodyJsonV5(@RequestBody HelloData helloData) throws IOException {
log.info("username={}, age={}",helloData.getUsername(),helloData.getAge());
return helloData;
}
์๋ต์ ๊ฒฝ์ฐ์๋ @ResponseBody ๋ฅผ ์ฌ์ฉํ๋ฉด ํด๋น ๊ฐ์ฒด๋ฅผ HTTP ๋ฉ์์ง ๋ฐ๋์ ์ง์ ๋ฃ์ด์ค ์ ์๋ค.
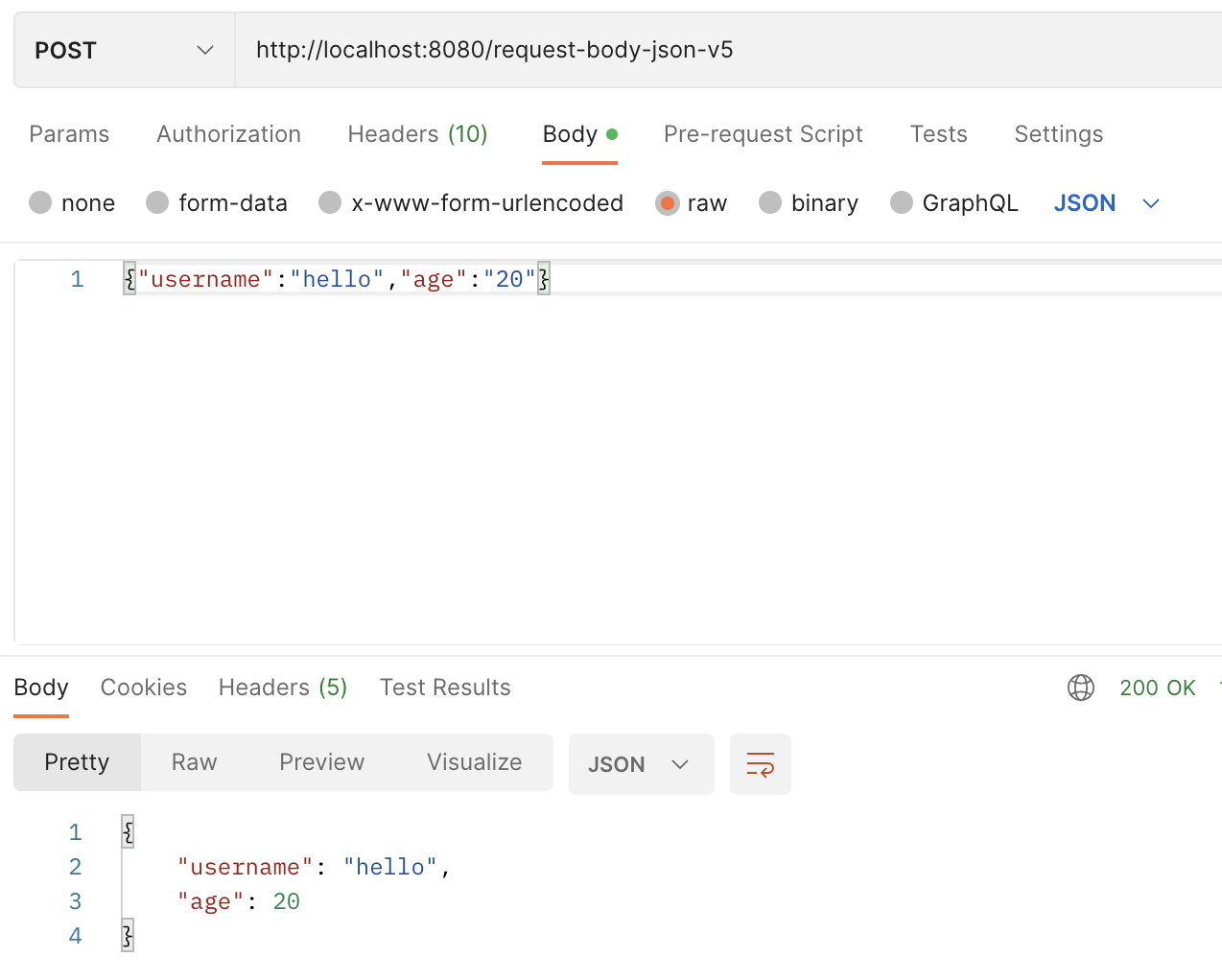
@RequestBody ์์ฒญ
JSON ์์ฒญ -> HTTP ๋ฉ์์ง ์ปจ๋ฒํฐ -> ๊ฐ์ฒด
@ResponseBody ์๋ต
๊ฐ์ฒด -> HTTP ๋ฉ์์ง ์ปจ๋ฒํฐ -> JSON ์๋ต
https://www.inflearn.com/course/%EC%8A%A4%ED%94%84%EB%A7%81-mvc-1/dashboard
์คํ๋ง MVC 1ํธ - ๋ฐฑ์๋ ์น ๊ฐ๋ฐ ํต์ฌ ๊ธฐ์ - ์ธํ๋ฐ | ๊ฐ์
์น ์ ํ๋ฆฌ์ผ์ด์ ์ ๊ฐ๋ฐํ ๋ ํ์ํ ๋ชจ๋ ์น ๊ธฐ์ ์ ๊ธฐ์ด๋ถํฐ ์ดํดํ๊ณ , ์์ฑํ ์ ์์ต๋๋ค. ์คํ๋ง MVC์ ํต์ฌ ์๋ฆฌ์ ๊ตฌ์กฐ๋ฅผ ์ดํดํ๊ณ , ๋ ๊น์ด์๋ ๋ฐฑ์๋ ๊ฐ๋ฐ์๋ก ์ฑ์ฅํ ์ ์์ต๋๋ค., -
www.inflearn.com
'Spring > Spring MVC' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[์คํ๋ง MVC] - 16. Thymeleaf ํ์๋ฆฌํ ์ฌ์ฉ๋ฒ ๋ฐ ๋ฌธ๋ฒ (0) | 2023.02.18 |
---|---|
[์คํ๋ง MVC] - 15. HTTP ์๋ต / HttpMessageConverter / ArgumentResolver (0) | 2023.02.17 |
[์คํ๋ง MVC] - 13. ์์ฒญ ๋งคํ / ์์ฒญ ํ๋ผ๋ฏธํฐ (0) | 2023.02.16 |
[์คํ๋ง MVC] - 12. ๋ก๊ทธ / SLF4J / Logback (0) | 2023.02.16 |
[์คํ๋ง MVC] - 11. @Controller / @RequestMapping (0) | 2023.02.15 |